Java | Operators | Expression
Java operators are symbols that perform operations on variables and values. They are used to manipulate data and perform mathematical or logical operations. Java operators can be classified into several categories:
Arithmetic Operators: Arithmetic operators are used to perform basic mathematical operations. They include addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
Addition (+): Adds two operands.
Subtraction (-): Subtracts the right operand from the left operand.
Multiplication (*): Multiplies two operands.
Division (/): Divides the left operand by the right operand.
Modulus (%): Returns the remainder of the division operation.
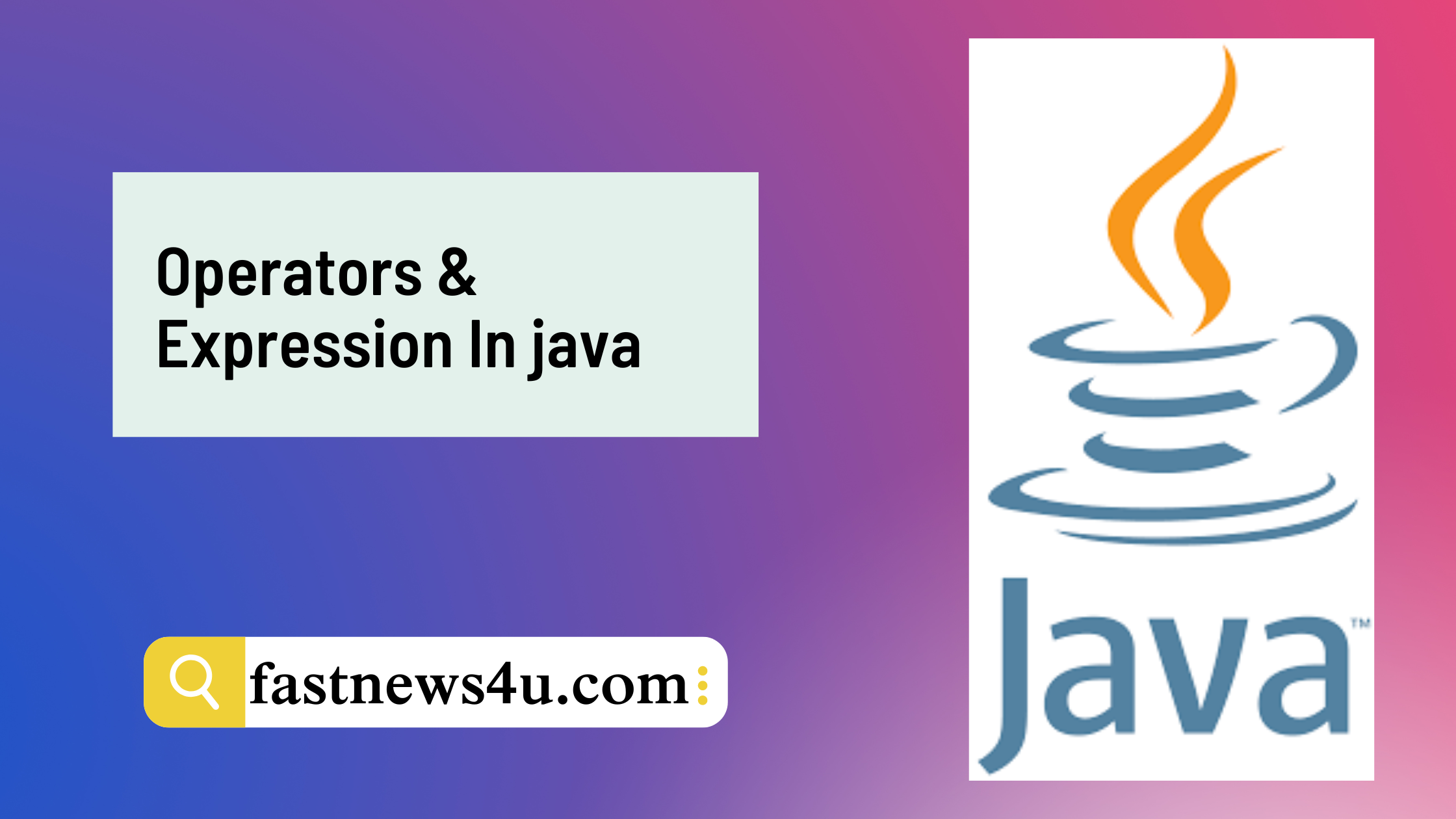
Assignment Operators: Assignment operators are used to assign values to variables. The basic assignment operator is “=”. For example, “int x = 10;” assigns the value 10 to the variable x.
Other assignment operators include +=, -=, *=, /=, and %=, which perform the operation and then assign the result to the variable. For example, “x += 5;” is equivalent to “x = x + 5;”.
Comparison Operators: Comparison operators are used to compare two values. They include == (equal to), != (not equal to), > (greater than), < (less than), >= (greater than or equal to), and <= (less than or equal to).
Logical Operators: Logical operators are used to perform logical operations. They include && (logical AND), || (logical OR), and ! (logical NOT). These operators are used to combine two or more conditions.
Bitwise Operators: Bitwise operators are used to perform bitwise operations on integer operands. They include & (bitwise AND), | (bitwise OR), ^ (bitwise XOR), ~ (bitwise complement), << (left shift), and >> (right shift).
Increment and Decrement Operators: Increment (++) and decrement (–) operators are used to increase or decrease the value of a variable by 1, respectively. They can be used as prefix (++x) or postfix (x++) operators.
Conditional (Ternary) Operator: The conditional operator (?:) is a ternary operator that evaluates a boolean expression and returns one of two values based on the result of the evaluation.
Instanceof Operator: The instanceof operator is used to test whether an object is an instance of a particular class or interface. It returns true if the object is an instance of the specified class or interface, otherwise false.
Null Coalescing Operator: The null coalescing operator (??) is used to return the left-hand operand if the operand is not null, otherwise it returns the right-hand operand.
Other Operators: Java also includes the instance of operator for type comparisons, the new operator for object instantiation, and the [] operator for array element access.
Expressions:
Expressions in Java are combinations of operators, variables, and literals that evaluate to a single value. For example, in the expression “int result = 5 + 3 * 2;”, the operators are “+” and “*”, and the variables are “5” and “3”. The expression evaluates to “11”, which is then assigned to the variable “result”.
Pingback: Control statements in java - Fast News4U